외부 엔코더 증감에 의해 위치가 이동하는 대상에 레이저 가공을 위해서는 특정 엔코더값에 도달할 때 까지 대기 후 가공을 시작하는 등 다양한 조건(Condition)을 이용하여 가공이 가능해야 합니다.
(참고) 이런 기능을 통상 MoF(Marking on the fly) 라고 합니다. (혹은 Processing on the fly)
이에 적합한 데모를 제공하고자 EDITOR_MOF 프로젝트가 제공됩니다. 여기에서는 MoF 시작, 끝 위치를 지정하고 특정 위치 (엔코더 값)를 대기하는등의 예를 보여주고 있습니다.
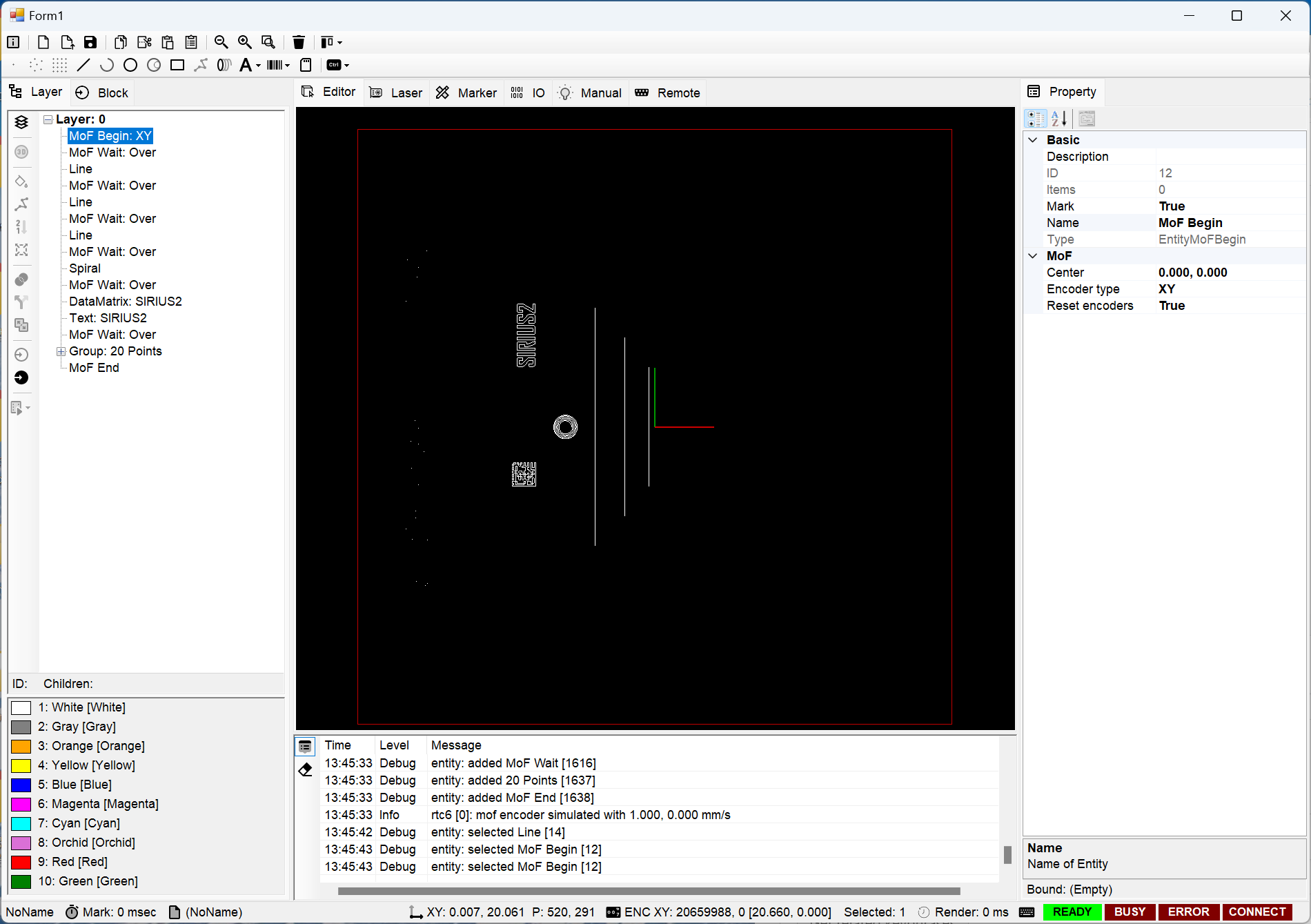
데모 프로젝트에서는 직접 코드를 사용해 다양한 개체(Entity) 들을 생성해 사용하고 있습니다.
(참고) 제어 개체(Control)생성 메뉴를 통해 프로그램 코드 없이 사용자가 직접 생성 가능합니다.
MoF 시작 제어 개체 생성
var mofBegin = EntityFactory.CreateMoFBegin(RtcEncoderTypes.XY, true);
document.ActAdd(mofBegin);
첫 번째 선분 개체 생성
double x1 = -1;
var mofWait1 = EntityFactory.CreateMoFWait(RtcEncoders.EncX, RtcEncoderWaitConditions.Over, -x1);
document.ActAdd(mofWait1);
var line1 = EntityFactory.CreateLine(x1, 10, x1, -10);
document.ActAdd(line1);
두 번째 선분 개체 생성
// 2nd line entity
double x2 = -5;
var mofWait2 = EntityFactory.CreateMoFWait(RtcEncoders.EncX, RtcEncoderWaitConditions.Over, -x2);
document.ActAdd(mofWait2);
var line2 = EntityFactory.CreateLine(x2, 15, x2, -15);
document.ActAdd(line2);
세 번째 선분 개체 생성
// 3rd line entity
double x3 = -10;
var mofWait3 = EntityFactory.CreateMoFWait(RtcEncoders.EncX, RtcEncoderWaitConditions.Over, -x3);
document.ActAdd(mofWait3);
var line3 = EntityFactory.CreateLine(x3, 20, x3, -20);
document.ActAdd(line3);
4번째 나선 개체 생성
// Spiral entity
double x4 = -15;
var mofWait4 = EntityFactory.CreateMoFWait(RtcEncoders.EncX, RtcEncoderWaitConditions.Over, -x4);
document.ActAdd(mofWait4);
var spiral = EntityFactory.CreateSpiral(x4, 0, 2, 4, 0, 5, true);
document.ActAdd(spiral);
5번째 바코드, 텍스트 개체 생성
double x5 = -20;
var mofWait5 = EntityFactory.CreateMoFWait(RtcEncoders.EncX, RtcEncoderWaitConditions.Over, -x5);
document.ActAdd(mofWait5);
var dataMatrix = EntityFactory.CreateDataMatrix("SIRIUS2", Barcode2DCells.Outline, 3, 4, 4);
dataMatrix.RotateZ(90);
dataMatrix.Name = "MyBarcode1";
dataMatrix.Translate(x5, -10);
document.ActAdd(dataMatrix);
var text = EntityFactory.CreateText(SpiralLab.Sirius2.Winforms.Config.InstalledFontNames[0], "SIRIUS2", FontStyle.Bold, 4);
text.RotateZ(90);
text.Translate(x5, 10);
document.ActAdd(text);
6번째 점 집합 개체 생성
double x6 = -40;
var mofWait6 = EntityFactory.CreateMoFWait(RtcEncoders.EncX, RtcEncoderWaitConditions.Over, -x6);
document.ActAdd(mofWait6);
var mofAndPointsList = new List<IEntity>(100);
double xRange = 2;
double yRange = 30;
var rnd = new Random();
for (int i = 0; i < 20; i++)
{
double x = x6 + rnd.NextDouble() * (xRange + xRange) - xRange;
double y = rnd.NextDouble() * (yRange + yRange) - yRange;
var point = EntityFactory.CreatePoint(new Vector2((float)x, (float)y), 20);
mofAndPointsList.Add(point);
}
var group = EntityFactory.CreateGroup("20 Points", mofAndPointsList.ToArray());
document.ActAdd(group);
MoF 끝 제어 개체 생성
var mofEnd = EntityFactory.CreateMoFEnd(Vector2.Zero);
document.ActAdd(mofEnd);
외부 엔코더 신호를 수신하기 위한 외부 배선 처리가 되어 있지 않더라도, 시리우스 라이브러리에서는 가상 엔코더 발생기능을 지원합니다. 이를 사용하기 위해서 아래와 같이 X 축용 엔코더를 1mm/s 속도로 발생시키게 됩니다.
var rtcMoF = rtc as IRtcMoF;
rtcMoF.CtlMofEncoderSpeed(1, 0);
위와 같이 코드상으로 설정이 가능하며, 또한 아래와 같이 레이저 탭에서 직접 변경도 가능합니다. 또한 해당 값이 설정되는 순간 엔코더 펄스 수 및 누적된 이동량이 하단의 엔코더 상태값에 지속적으로 업데이트 되어 출력 됩니다.
(참고) 하단 엔코더 값을 더블클릭시 0 으로 리셋됩니다
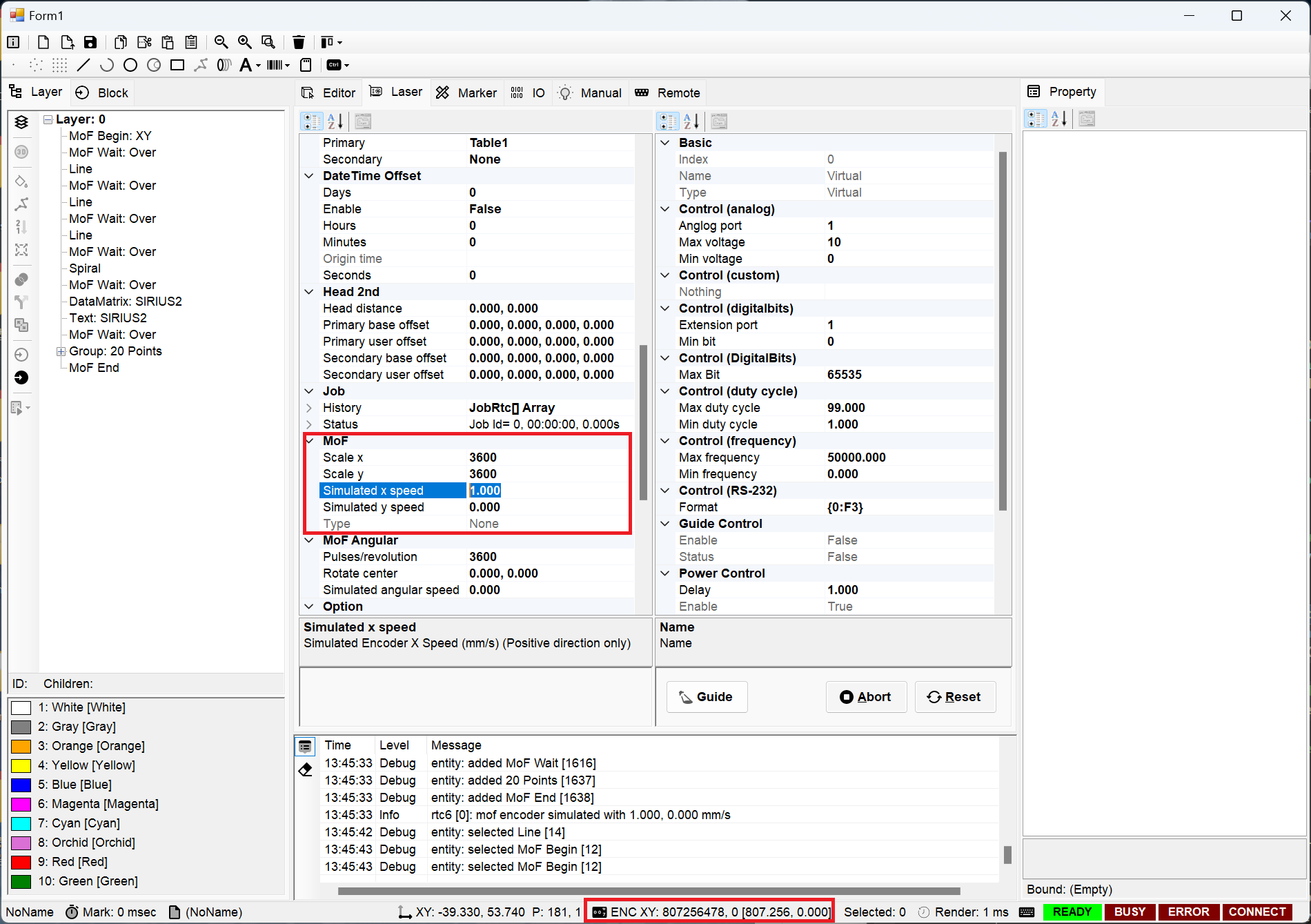
예를 들어 20개의 점 개체의 가공 시점은, 개체 앞에 추가된 MoF Wait 제어 개체에 의해 엔코더 X 의 누적값이 40mm가 넘을 때(Over)까지 대기 후 이를 초과하면 엔코더 증값값이 추가되면서 20개의 점 개체들이 가공됩니다.
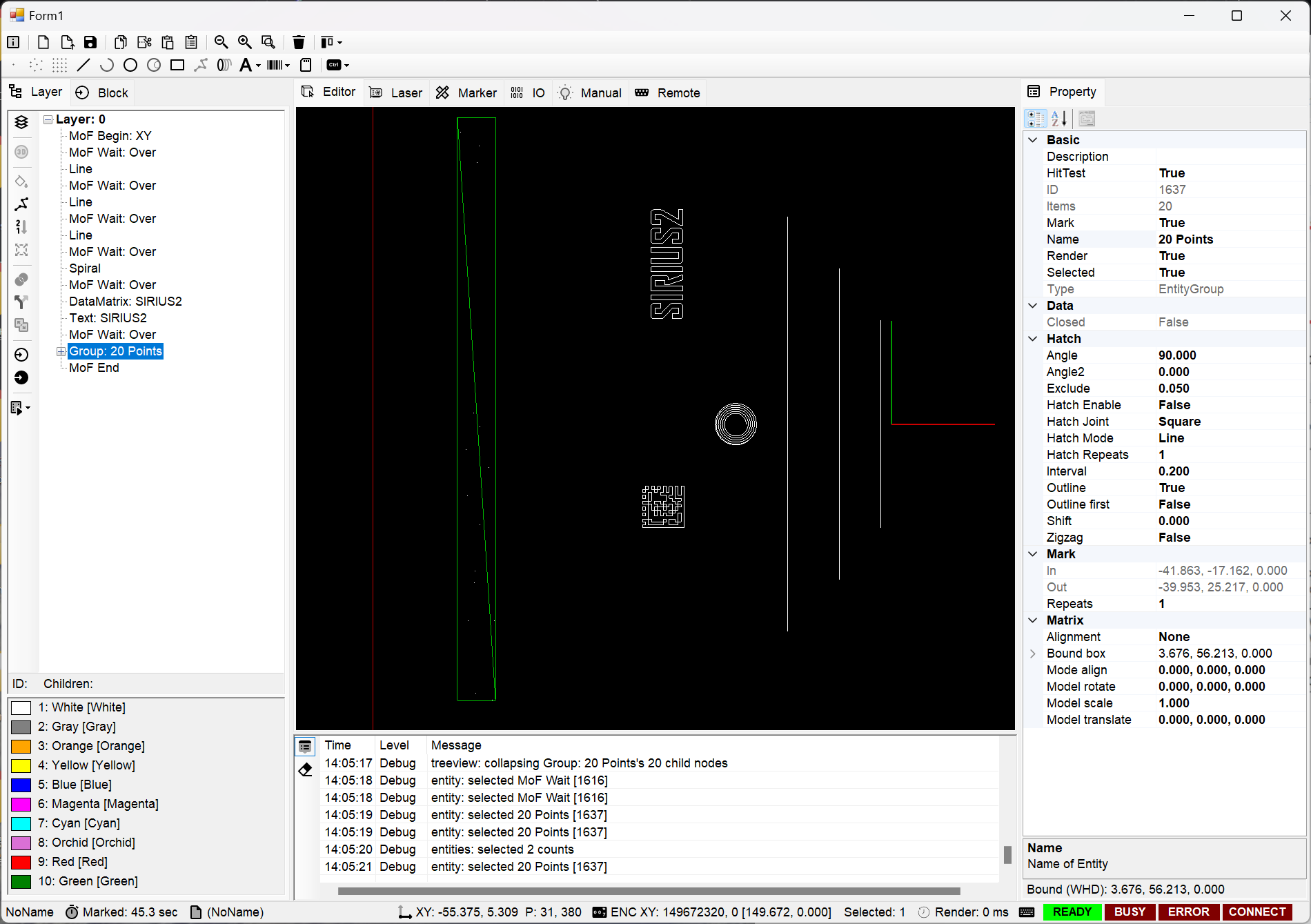